Native Integration Guide
This document provides a step by step guide on how to install, integrate and initialize the BlockID SDK into any Android applications.
Prerequisites
There are a few general and platform-specific dependencies required to integrate BlockID SDK into an Android application. Developers should take note of the following requirements:
General
- An understanding of how to develop applications for Android
- At least one compatible Android mobile device
Technical
- Android API Level 23 (Mashmallow) / Android OS 6.0 or higher
- Android Studio Chipmunk | 2021.2.1
- Device support - all devices running Android OS 6.0 and higher
The BlockID SDK currently supports up to Android OS 13.x
Get Started immediately
Please request access to our SDK and demo applications by sending us an email at developers@1kosmos.com
Developers can request access to the Android BlockID Mobile SDK and Demo Application to access the code and see how the BlockID SDK can be used.
Integration Overview
This section explains the step by step guide on how to setup and integrate the BlockID SDK in an Android application.
The examples provided below demonstrate the integration steps of the BlockID SDK into a new Android project
The same steps can also be used for integration into an existing application
Screenshots in this section are generated using Android Studio Bumblebee for macOS
Step 1: Create a New Application
- Open
Android Studio
- From the Welcome Screen, click New Project
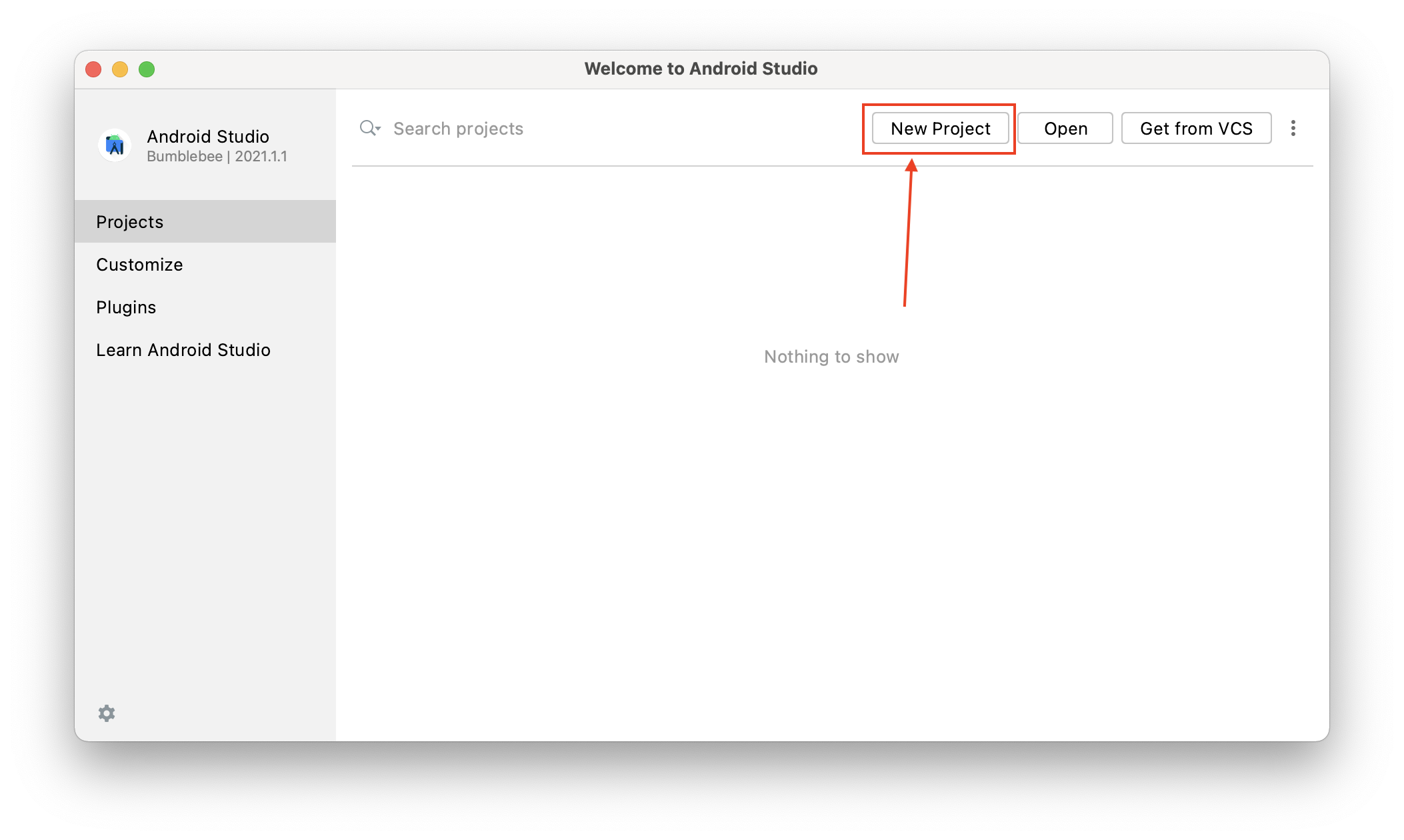
- From the New Project screen, select a template and press Next
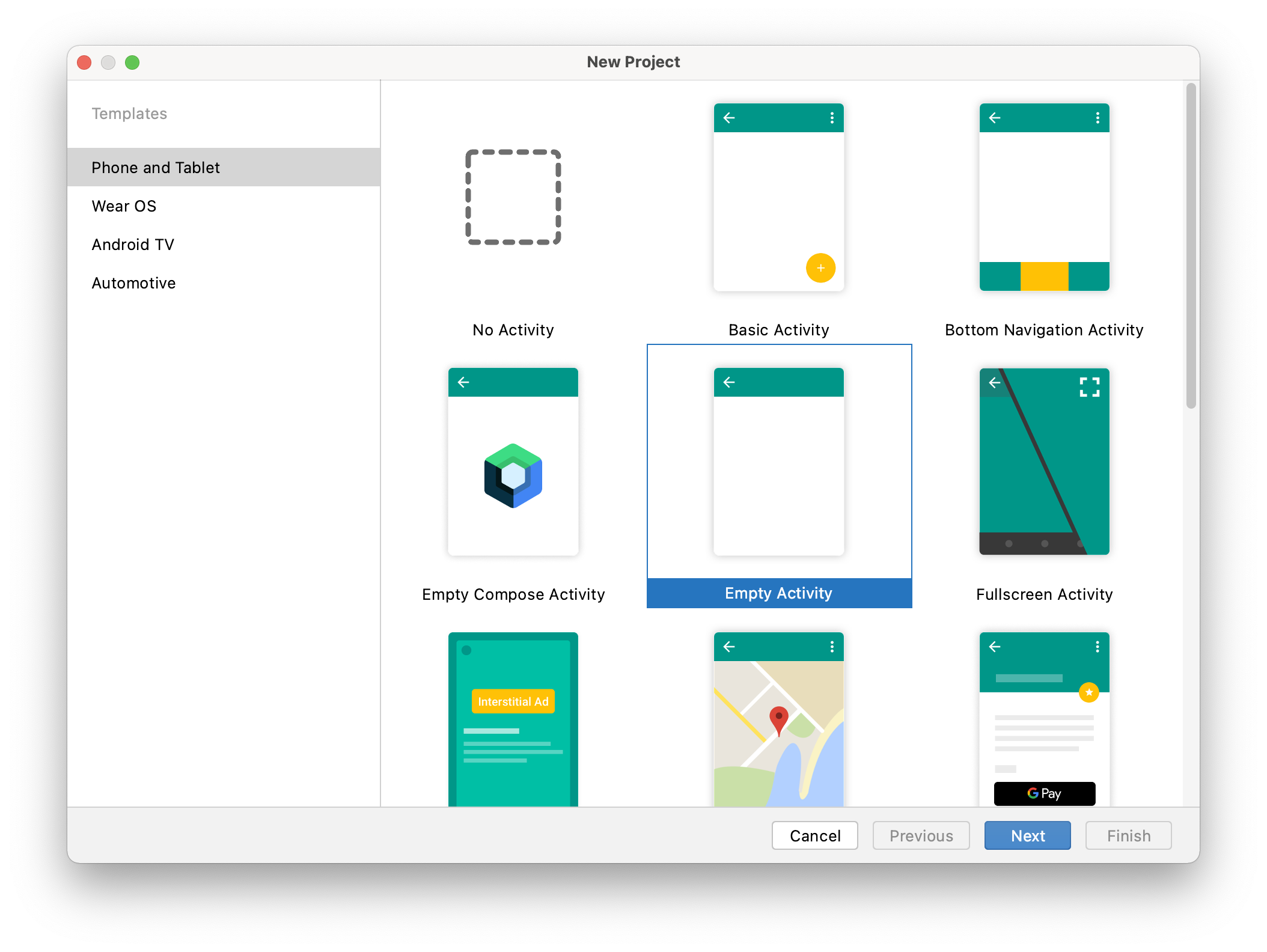
- Enter a project name, such as BlockID Demo
- Update any additional parameters, such as location or language (optional)
- Set the Minimum SDK to API 23: Android 6.0 (Mashmallow)
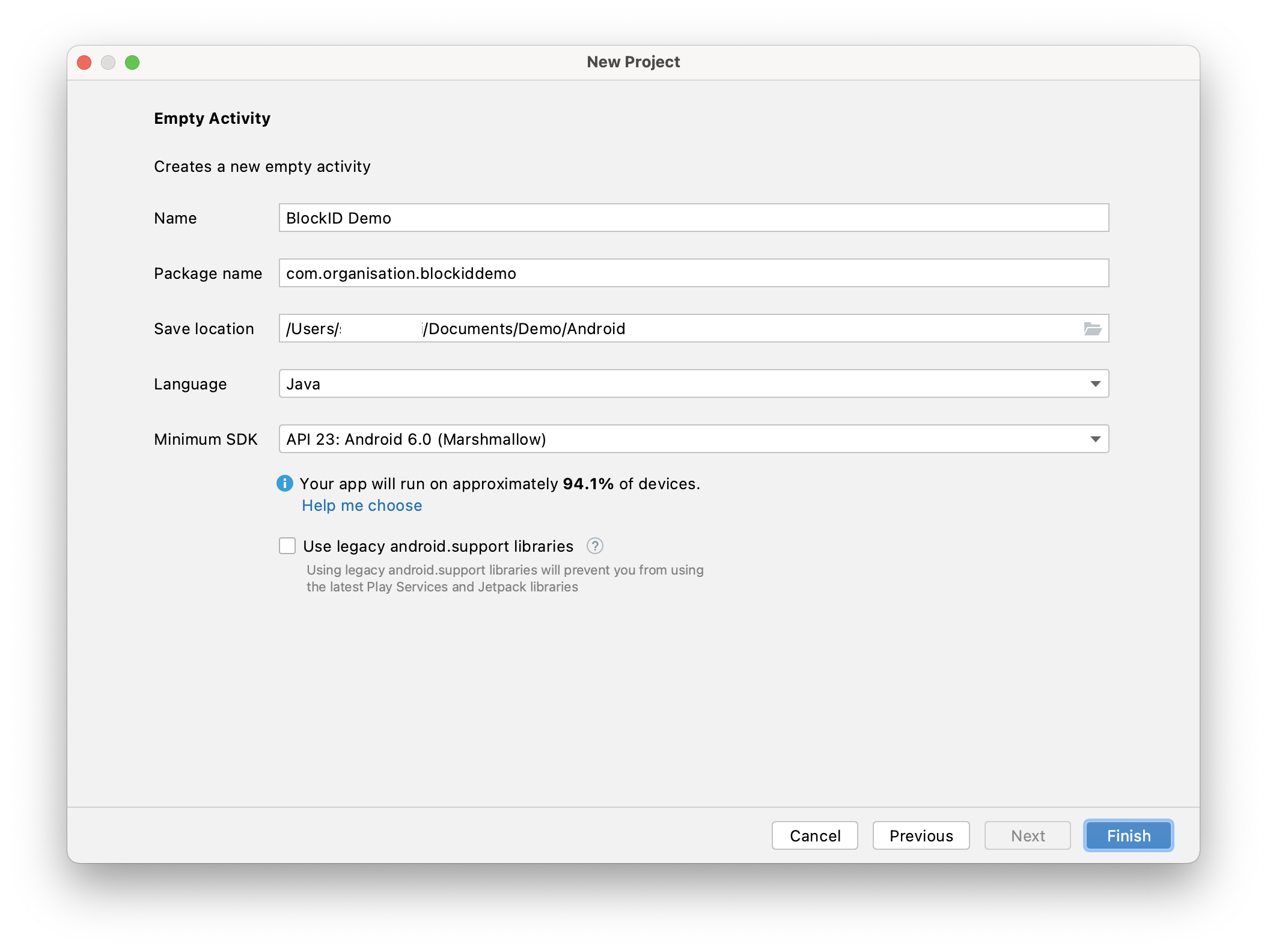
- Click Finish and wait a few moments for the project to finish configuring itself
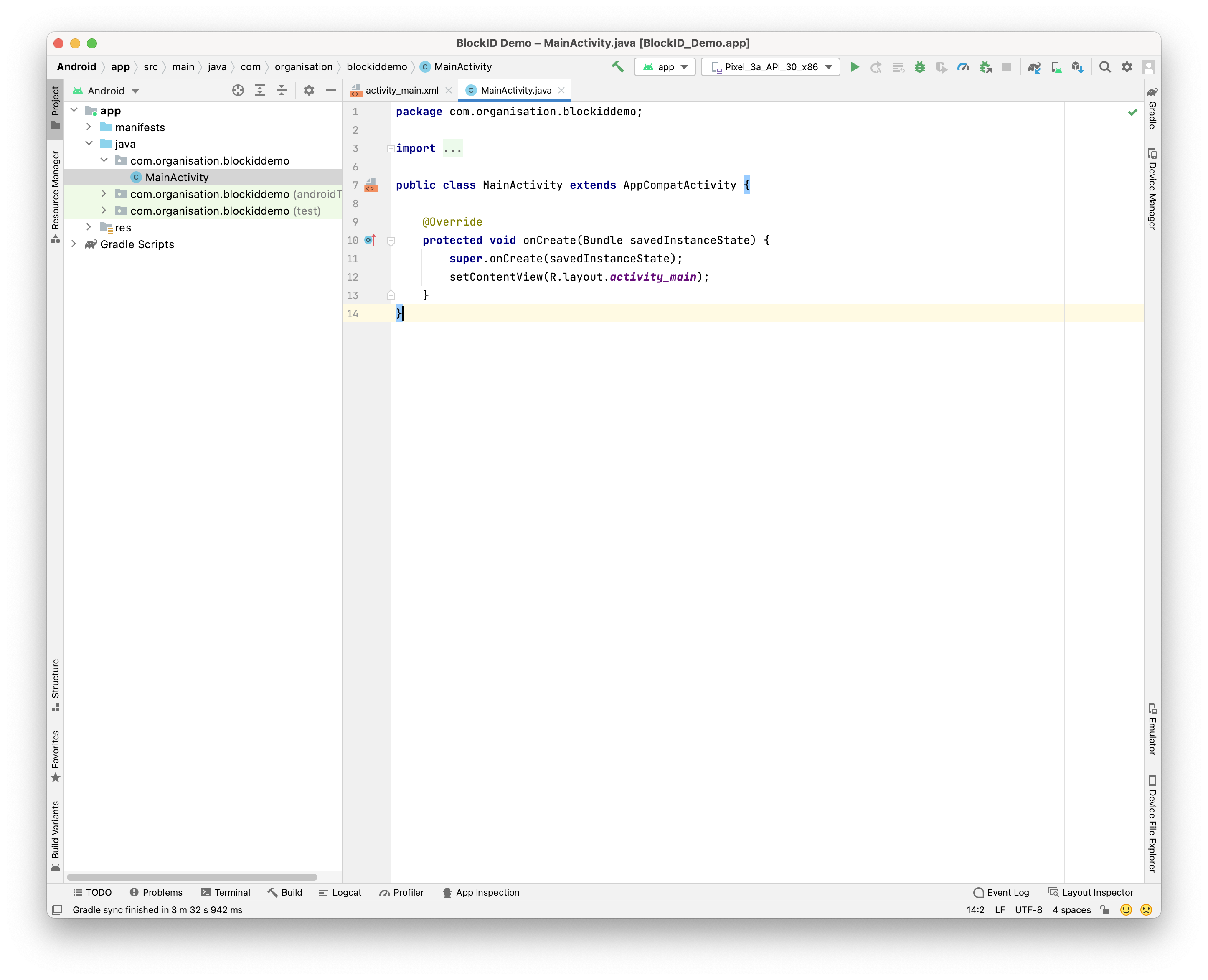
Step 2: Add BlockID SDK
Now that our project has been created, we can add the BlockID SDK as a gradle
dependency.
- Navigate to your Android Studio project and open the app level
build.gradle
file. Add the line shown below to yourbuild.gradle
:
// Add BlockIDSDK dependency
implementation 'com.onekosmos.blockid.sdk:blockidsdk:1.9.40.6495ABDF'
- Next, open your
settings.gradle
file and add the following block:
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
//noinspection JcenterRepositoryObsolete
jcenter()
mavenCentral()
maven {
url 'https://nexus-1k-nonprod.1kosmos.net/repository/maven-releases/'
credentials {
username = "developer"
password = "q5k#06ZcjSo#"
}
}
maven { url "https://jitpack.io" }
maven {
url 'http://www.baka.sk/maven2'
allowInsecureProtocol = true
}
}
}
Step 3: Java Compatibility
The BlockID SDK is not binary compatible with applications that target Java 8
In order to use the SDK you must upgrade your applications to target Java 11.
Below is a snippet for reference:
android {
compileOptions {
sourceCompatibility JavaVersion.VERSION_11
targetCompatibility JavaVersion.VERSION_11
}
}
Step 4: Update Android Manifest
The BlockID SDK provides scanning features for various documents for which the application must enable and ask for permission to use. To be compatible with Android API Version 23 and later, you will need to ensure that your application is set to request runtime permissions for the camera (and others per project requirements).
To enable camera permissions, the following steps must be taken.
4.1 Update Application Tag
BlockID SDK ensures not to share data outside of its own enclave. Application developers must add the attribute shown below to the <application>
tag in the application's manifest file:
tools:replace="android:allowBackup,android:label,android:theme"
4.2 Add Permissions in Android Manifest
Next, we need to add some permissions to the Android manifest.
Add the following codeblock to your application AndroidManifest.xml
:
// Add camera permission
<uses-permission android:name="android.permission.CAMERA" />
// Add NFC permission for RFID Scanning
<uses-permission android:name="android.permission.NFC" />
// Additional permissions
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
4.3 Request Camera Permission
Request camera permissions within your application code:
ActivityCompat.requestPermissions(this, new String[] { Manifest.permission.{your_permission}, PERMISSION_REQUEST_CODE );
Step 5: Add Gradle Dependencies
The BlockID SDK has dependencies from other libraries it uses. You will need to add those dependencies to your project.
5.1 Application Level Dependencies
The following section contains all the dependencies (along with their respective purpose) that need to be added at the application level build.gradle
file:
// Gson
implementation 'com.google.code.gson:gson:2.9.0'
// secure shared preference
implementation 'androidx.security:security-crypto:1.1.0-alpha03'
// QR code scan and Driver's License scanning
implementation 'com.google.android.gms:play-services-vision:20.1.3'
// wallet
implementation 'org.web3j:core:4.8.7-android'
// generate mnemonics
implementation('org.bitcoinj:bitcoinj-core:0.16.1') {
exclude group: 'com.google.protobuf', module: 'protobuf-java'
exclude group: 'net.jcip', module: 'jcip-annotations'
}
// fingerprint and biometric
implementation 'androidx.biometric:biometric:1.1.0'
// annotations
implementation 'org.jetbrains:annotations:23.0.0'
// base32 encode/decode
implementation 'commons-codec:commons-codec:1.11'
// network call
implementation 'com.amitshekhar.android:android-networking:1.0.2'
// passport scan
implementation('org.jmrtd:jmrtd:0.7.32') {
exclude module: 'bcprov-jdk15on'
exclude module: 'bctls-jdk15on'
}
// mrz scan
implementation 'org.innovatrics.mrz:mrz-java:0.4'
// RFID scan
implementation 'androidx.multidex:multidex:2.0.1'
implementation 'net.sf.scuba:scuba-sc-android:0.0.23'
implementation 'com.madgag.spongycastle:prov:1.58.0.0'
implementation 'com.github.mhshams:jnbis:1.1.0'
implementation 'com.gemalto.jp2:jp2-android:1.0'
// filter document
implementation 'com.annimon:stream:1.2.2'
// CameraX
implementation 'androidx.camera:camera-camera2:1.2.0-alpha03'
implementation 'androidx.camera:camera-lifecycle:1.2.0-alpha03'
implementation 'androidx.camera:camera-view:1.2.0-alpha03'
// LiveID
implementation 'com.google.android.gms:play-services-mlkit-face-detection:17.0.1'
implementation 'com.google.guava:guava:31.1-jre'
// text recognition
implementation 'com.google.android.gms:play-services-mlkit-text-recognition:18.0.0'
// barcode detector
implementation 'com.google.mlkit:barcode-scanning:17.0.2'
// image processing
implementation 'com.quickbirdstudios:opencv:4.5.3.0'
// custom tab intent
implementation 'androidx.browser:browser:1.4.0'
// Walletconnect
implementation 'com.walletconnect:sign:2.0.0-rc.5'
implementation 'com.walletconnect:android-core:1.0.0'
implementation 'org.web3j:utils:5.0.0'
// Native FIDO2 External
implementation 'com.google.android.gms:play-services-fido:19.0.0'
implementation 'androidx.lifecycle:lifecycle-process:2.5.1'
Add the following block to resolve bouncycastle
conflicts:
android {
...
}
configurations.all {
resolutionStrategy.eachDependency { DependencyResolveDetails details ->
if (details.requested.group == 'org.bouncycastle') {
details.useTarget 'org.bouncycastle:bcprov-jdk15to18:1.70'
}
}
}
Add the following block to resolve META-INF
conflicts:
android {
...
packagingOptions {
exclude 'META-INF/rxjava.properties'
exclude 'lib/x86_64/darwin/libscrypt.dylib'
exclude 'lib/x86_64/freebsd/libscrypt.so'
exclude 'lib/x86_64/linux/libscrypt.so'
exclude 'META-INF/INDEX.LIST'
exclude 'META-INF/LICENSE.md'
exclude 'META-INF/DEPENDENCIES'
exclude 'META-INF/NOTICE.md'
exclude 'AndroidManifest.xml'
}
}
Step 6: Enable Proguard
If you wish to obfuscate your application code, create or edit your proguard-rule.pro
file and include the following lines:
-keep class com.onekosmos.blockid.sdk.** { *; }
-keep public class org.** { *; }
# walletconnect
-keep class com.walletconnect.**{* ;}
-keep class net.sqlcipher.** { *; }
-keep class net.sqlcipher.database.** { *; }
Step 7: Gradle Properties
Add the line below to your gradle.properties
file:
android.enableJetifier=true
Step 8: Application Deployment
Deploying your Android application once integrated with the BlockID SDK does not require any additional steps to your deployment process.
Initial SDK Setup
The BlockID SDK should now be fully added to your application at this point.
In order to use the features of the BlockID SDK, you must add your License Key, Wallet, and Tenant details. This information will be provided with SDK access.
Please contact us at developers@1kosmos.com for access to the SDK
The credentials listed on your Developer Dashboard will not work with the BlockID Mobile SDK
The information must be set using following sequence only and MUST NOT be changed
Step 1: Initialize SDK
The BlockID SDK must be initialized to ensure that all its key components are setup and working. Any other SDK functions can only be used once the initialization process has been completed.
Add the following line to your application code to initialize the BlockID SDK:
BlockIDSDK.initialize(<the_context>);
Step 2: Set License Key
The license key must be set on every launch of the application
Whenever the set license key method is called the SDK internally checks if:
- the given license key is valid
- the application is authorized to use the given license key
Add the following code to your application to set the license key:
BlockIDSDK.getInstance().setLicenseKey("<your_license_key>")
Step 3: Initialize Wallet
Before registering tenant, a temporary application wallet must be created. An application can only have one wallet, hence the temporary wallet must be created ONLY once.
Add the following code to initialize a temporary wallet:
BlockIDSDK.getInstance().initiateWallet();
Step 4: Register Tenant
4.1 About Tenant
The tenant can be represented as the value or an object on which API calls can be registered.
There are two types of tenants:
- Root Tenant: stores details about enrolled user biometric informatiom and digital assets
- Client Tenant: stores user's enrolled persona
A root tenant can also store a user's persona, but a client tenant cannot be used to store biometric data or digital assets
The application is expected to register a tenant only once. If an existing tenant is set, the application will load it after validating the license key.
The SDK will only allow a tenant to be registered after the license key is set.
The tenant object is represented using the BIDTenant
class, and can be created using the following code:
BIDTenant bidTenant = new BIDTenant("<tenant tag>", "<community name>", "<tenant DNS>");
4.2 Register Tenant
After the object BIDTenant
is created the tenant registration API must be called:
BlockIDSDK.getInstance().registerTenant(bidTenant, BIDTenantRegistryCallback);
The tenant details will be used while enrolling documents, or when getting a public key. When this function is executed, an Ethereum wallet is created and a Distributed Identifier (DID), along with 12 recovery Mnemonic phrases will be avaible to the user.
Step 5: Commit Wallet
Upon successful tenant registration, the commit wallet method must be called. The method must be called only once:
BlockIDSDK.getInstance().commitApplicationWallet();
The commit wallet method is the final step for setting up BlockID SDK into an Android application.
SDK Security
Because the attack surface of an API is wider than that of a standlone application. the BlockID SDK has implemented a locking mechanism to prevent any kind of unauthorized access.
For security purposes, the points below must be implemented in the application:
- SDK should be locked when the application moves into background states
- SDK should be locked when application shows login screen to Users
- SDK lock status should be checked before calling any enrollment APIs
Refer to the API Reference Guide for Android for more details on the methods used in this section