Poll UWL Session
Poll a UWL Session
When a session is created using the SDK, a unique session ID and URL endpoint are created, and a polling service starts looking for a response.
The session will remain valid for 60 seconds. If the polling service does not receive a response within that timeframe, the session will expire and close.
To authenticate the request using the BlockID mobile application you will need to encode the session information in a QR code, such as the one in the image below.
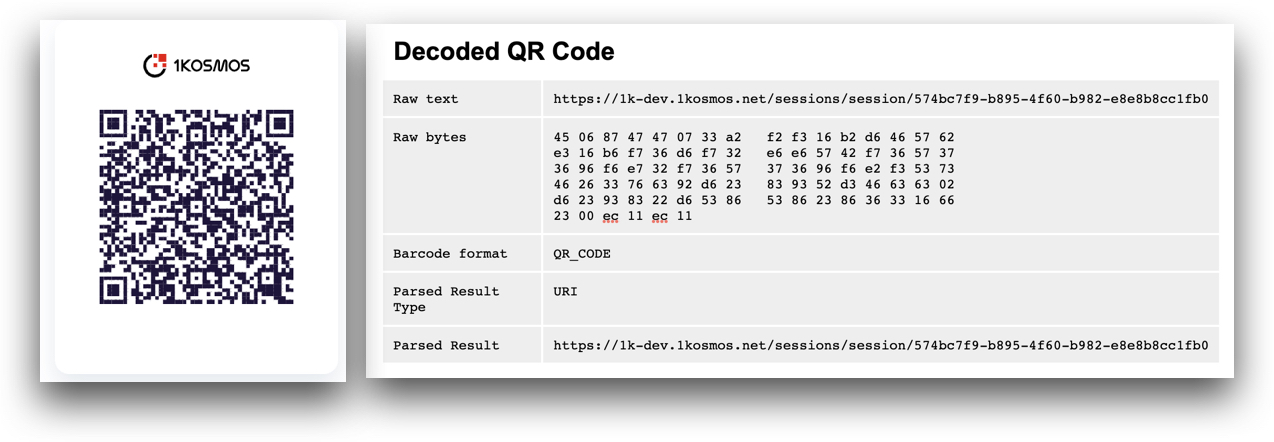
Parameters
dns
: Your BlockID tenant domain as shown in the dashboardcommunityName
(required): Your BlockID tenant community as shown in the dashboardlicenseKey
(required): BlockID license key as shown in the dashboardsessionId
: UWL session ID string (required)
Request Format
- NodeJS SDK
- PHP SDK
- Java SDK
- .NET SDK
- Set tenant info and poll for UWL session response from the BlockID mobile application
const BIDSessions = require('blockid-nodejs-helpers/BIDSessions');
let authSessionRespone = await BIDSessions.pollSession({ "dns": "<dns>", "communityName": "<communityName>", "licenseKey": "<licenseKey>" }, "<sessionId>", true, true);
- Set tenant info and poll UWL session response
<?php
require_once("./BIDTenant.php");
require_once("./BIDSession.php");
$tenantInfo = array("dns" => "<dns>", "communityName" => "<communityName>", "licenseKey" => "<licenseKey>");
$pollSessionResponse = BIDSession::pollSession($tenantInfo, "<sessionId>", TRUE, TRUE);
?>
- Set tenant info and poll UWL session response
BIDTenantInfo tenantInfo = new BIDTenantInfo("<dns>", "<communityName>", "<license>");
BIDSessionResponse response = BIDSessions.pollSession(tenantInfo, "<sessionId>", true, true);
- Set tenant info and poll UWL session response
using BIDHelpers.BIDTenant.Model;
using BIDHelpers.BIDSessions;
using BIDHelpers.BIDSessions.Model;
BIDTenantInfo bidTenantInfo = new BIDTenantInfo("<dns>", "<communityName>", "<licenseKey>");
BIDSessionResponse pollResponse = BIDSessions.PollSession(bidTenantInfo, "<sessionResponse.sessionId>", true, true);
Example UWL Request
- NodeJS SDK
- PHP SDK
- Java SDK
- .NET SDK
Example - Poll UWL Session
Poll a UWL session for a response from the BlockID mobile application.
const BIDSessions = require('blockid-nodejs-helpers/BIDSessions');
let authSessionRespone = await BIDSessions.pollSession({ "dns": "blockid-trial.1kosmos.net", "communityName": "devx", "licenseKey": "0005c9f8-1918-40be-aa00-e319043f7xxx" }, "574bc7f9-b895-4f60-b982-e8e8b8cc1fb0", true, true);
Example - Poll UWL Session
Poll a UWL session for a response from the BlockID mobile application.
<?php
require_once("./BIDTenant.php");
require_once("./BIDSession.php");
$tenantInfo = array("dns" => "blockid-trial.1kosmos.net", "communityName" => "devx", "licenseKey" => "0005c9f8-1918-40be-aa00-e319043f7xxx");
$pollSessionResponse = BIDSession::pollSession($tenantInfo, "574bc7f9-b895-4f60-b982-e8e8b8cc1fb0", TRUE, TRUE);
?>
Example - Poll UWL Session
Poll a UWL session for a response from the BlockID mobile application.
BIDTenantInfo tenantInfo = new BIDTenantInfo("blockid-trial.1kosmos.net", "devx", "0005c9f8-1918-40be-aa00-e319043f7xxx");
BIDSessionResponse response = BIDSessions.pollSession(tenantInfo, "574bc7f9-b895-4f60-b982-e8e8b8cc1fb0", true, true);
Example - Poll UWL Session
Poll a UWL session for a response from the BlockID mobile application.
using BIDHelpers.BIDTenant.Model;
using BIDHelpers.BIDSessions;
using BIDHelpers.BIDSessions.Model;
BIDTenantInfo bidTenantInfo = new BIDTenantInfo("blockid-trial.1kosmos.net", "devx", "b65b22ec-181a-4b7f-8116-6eee2a00000");
BIDSessionResponse response = BIDSessions.PollSession(bidTenantInfo, sessionInfo.sessionId, true, true);
Example Server Response
The SDK will return an immediate response from our API once the session has been authenticated in the BlockID mobile application. The responses are the same across each SDK and are JSON formatted. Possible response codes you might receive include:
- 200 OK
- 400 Expired
- 404 Session Doesn't Exist
- 404 Not Found
{
sessionId: "96ea590d-2c5f-4eb9-8a75-d4d96d8e62c6",
data: "OGI4NDA3OTJiYThkNWUxYhaSGtXOdBHvtWg893fB8XJfrP4WAsQeWy+04FGyE/CozBhtzBQpfgC/TFVyXWPcTRNMho4QeIixirPqkC6I2+xxxx==",
appid: "com.onekosmos.kernel.blockid",
ial: "1",
publicKey:
"5Uqyd3RgsZPYsVoeuVVkGwK+X7QLB9N6rDgSFiUNyX1lZw4Hiu6imKXAed6HtLDRd1I1XZII7krNsQqdlu7FWQ==",
createdTS: 1667808572,
createdDate: "2022-11-07T08:09:32.507Z",
status: 200,
message: null,
user_data: {
did: "18f01772e4d47bf65ea60001211709637fb01xxx",
},
account_data: {
id: "623871d6e072603c9c84081f",
personId: "18f01772e4d47bf65ea60001211709637fb0xxxx",
userIdList: ["dummy@1kosmos.com"],
communityId: "5f3d8d0cd866fa61019cf969",
publicKey:
"5Uqyd3RgsZPYsVoeuVVkGwK+X7QLB9N6rDgSFiUNyX1lZw4Hiu6imKXAed6HtLDRd1I1XZII7krNsQqdlu7FWQ==",
poi_ial: "",
pon_ial: "",
device: {
uid: "c907298d53273143",
did: "18f01772e4d47bf65ea60001211709637fb010ca",
os: "android",
publickey:
"5Uqyd3RgsZPYsVoeuVVkGwK+X7QLB9N6rDgSFiUNyX1lZw4Hiu6imKXAed6HtLDRd1I1XZII7krNsQqdlu7FWQ==",
deviceName: "Vivo 1718",
authenticatorId: "com.onekosmos.kernel.blockid",
authenticatorVersion: "1.6.00.621E14F8",
authenticatorName: "BlockID",
userAgent:
"Mozilla/5.0 _Linux_ Android 8.1.0_ vivo 1718 Build/OPM1.171019.026_ wv_ AppleWebKit/537.36 _KHTML_ like Gecko_ Version/4.0 Chrome/105.0.5195.79 Mobile Safari/537.36",
locLat: "31.9431928",
locLon: "75.8046449",
id: "6232037a25e1fb255a4faf07",
},
message: null,
status: false,
},
};
The session was successfully polled and the BlockID mobile application returned a valid response.
{ status: 400, message: 'Session has expired' },
The API received an expired session ID.
{ status: 404, message: "Session with this sessionId doesn't exist" },
The API received a session ID that has already had a response returned from the BlockID mobile application.
Once a session has been created and the polling service receives a valid response from the BlockID mobile application, our API will close the session . A session ID can only be used once.
{ status: 404, message: 'Not Found' },
The API could not find the session. Typically this means an incorrect session ID was provided, or the API did not receive any response from the BlockID mobile application.