Verify One-Time Passcode
Use the BlockID SDK to verify the six-digit one-time passcode you received via SMS or email.
Verify One-Time Passcode
You can verify an OTP request immediately after receiving your six-digit one-time passcode.
Each one-time passcode is only valid for a single verification. Submitting an incorrect one-time passcode more than five times in 24 hours will result in a 30-minute account lockout.
Parameters
dns
: Your tenant domain as shown in the dashboardcommunityName
: Your tenant communnity name as shown in the dashboardlicenseKey
: Your tenant license key as shown in the dashboardusername
: BlockID usernameotpCode
: Six-digit one-time passcode you received
Verification Format
- NodeJS SDK
- PHP SDK
- Java SDK
- .NET SDK
- Set tenant info and verify OTP
const BIDOTP = require('blockid-nodejs-helpers/BIDOTP');
let verifyOtpResponse = await BIDOTP.verifyOTP({ "dns": "<dns>", "communityName": "<communityName>", "licenseKey": "<licenseKey>" }, "<username>", "<otpCode>");
- Set tenant info and verify OTP
<?php
require_once("./BIDTenant.php");
require_once("./BIDOTP.php");
$tenantInfo = array("dns" => "<dns>", "communityName" => "<communityName>", "licenseKey" => "<licenseKey>");
$verifyOTPResponse = BIDOTP::verifyOTP($tenantInfo, "<userId>", "<otpCode>");
?>
- Set tenant info and verify OTP
BIDTenantInfo tenantInfo = new BIDTenantInfo("<dns>", "<communityName>", "<licenseKey>");
BIDOtpVerifyResult result = BIDOTP.verifyOTP(tenantInfo, "<username>", "<otpCode>");
- Set tenant info and verify OTP
using BIDHelpers.BIDOTP;
using BIDHelpers.BIDOTP.Model;
using BIDHelpers.BIDTenant.Model;
BIDTenantInfo bidTenantInfo = new BIDTenantInfo("<dns>", "<communityName>", "<licenseKey>");
BIDOtpVerifyResult verifyOTP = BIDOTP.VerifyOTP(bidTenantInfo, "<username>", "<otpCode>");
Example OTP Verification
We've provided some example OTP verifications using the BlockID SDK.
Please note that these are just examples - a typical use-case would be to require verification of a one-time passcode as part of a sign-in request.
- NodeJS SDK
- PHP SDK
- Java SDK
- .NET SDK
Verify a one-time passcode request using the six digits you received via email or SMS.
Example OTP Request - SMS
Example SMS containing six-digit one-time passcode.
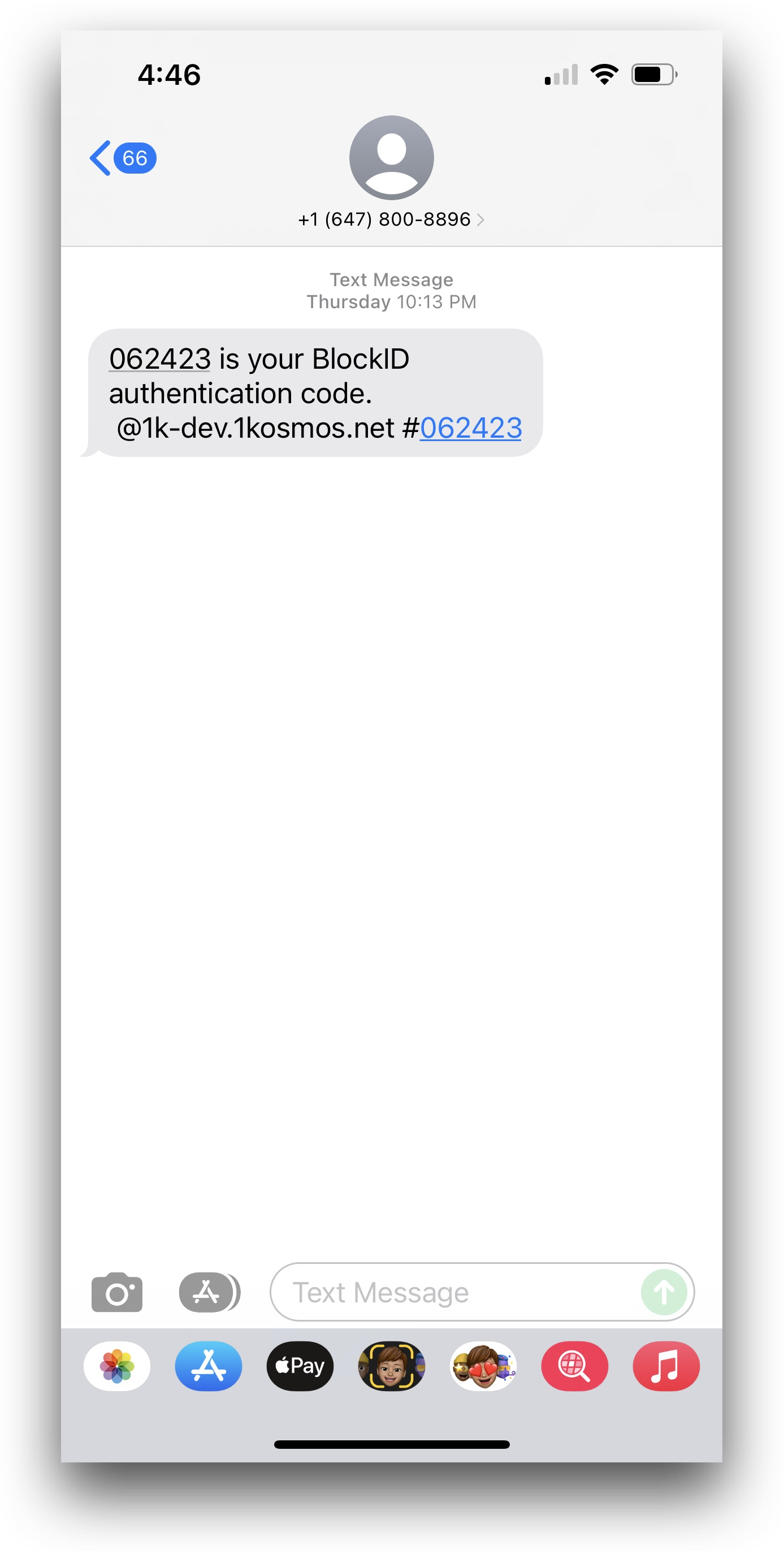
Example OTP Verifcation
const BIDOTP = require('blockid-nodejs-helpers/BIDOTP');
let verifyOtpResponse = await BIDOTP.verifyOTP({ "dns": "blockid-trial.1kosmos.net", "communityName": "devx", "licenseKey": "0005c9f8-1918-40be-aa00-e319043f7xxx" }, "john.smith@company.com", "062423");
Verify a one-time passcode request using the six digits you received via email or SMS.
Example OTP Request - SMS
Example SMS containing six-digit one-time passcode.
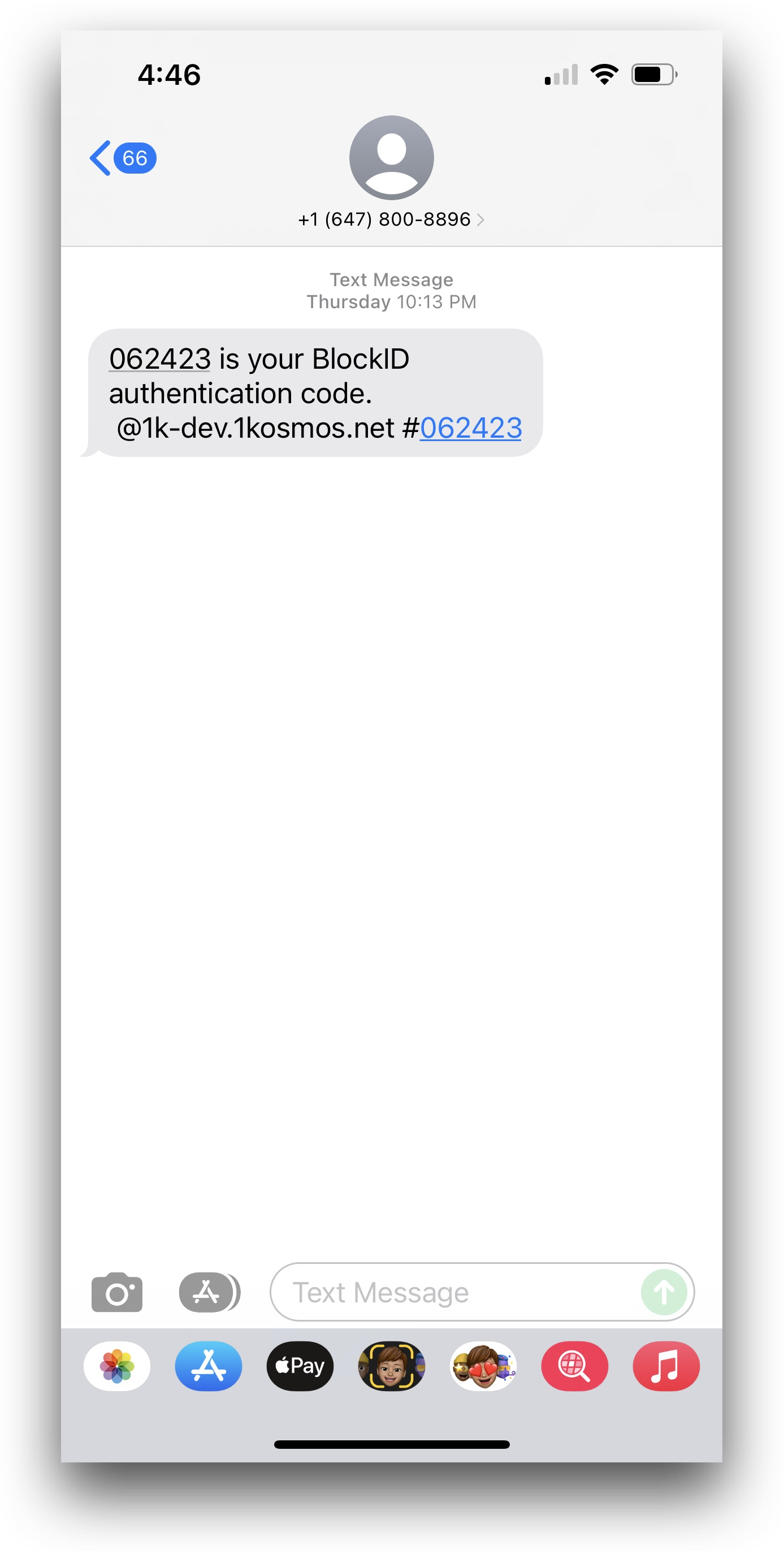
Example OTP Verifcation
<?php
require_once("./BIDTenant.php");
require_once("./BIDOTP.php");
$tenantInfo = array("dns" => "blockid-trial.1kosmos.net", "communityName" => "devx", "licenseKey" => "0005c9f8-1918-40be-aa00-e319043f7xxx");
$verifyOTPResponse = BIDOTP::verifyOTP($tenantInfo, "john.smith@company.com", "062423");
?>
Verify a one-time passcode request using the six digits you received via email or SMS.
Example OTP Request - SMS
Example SMS containing six-digit one-time passcode.
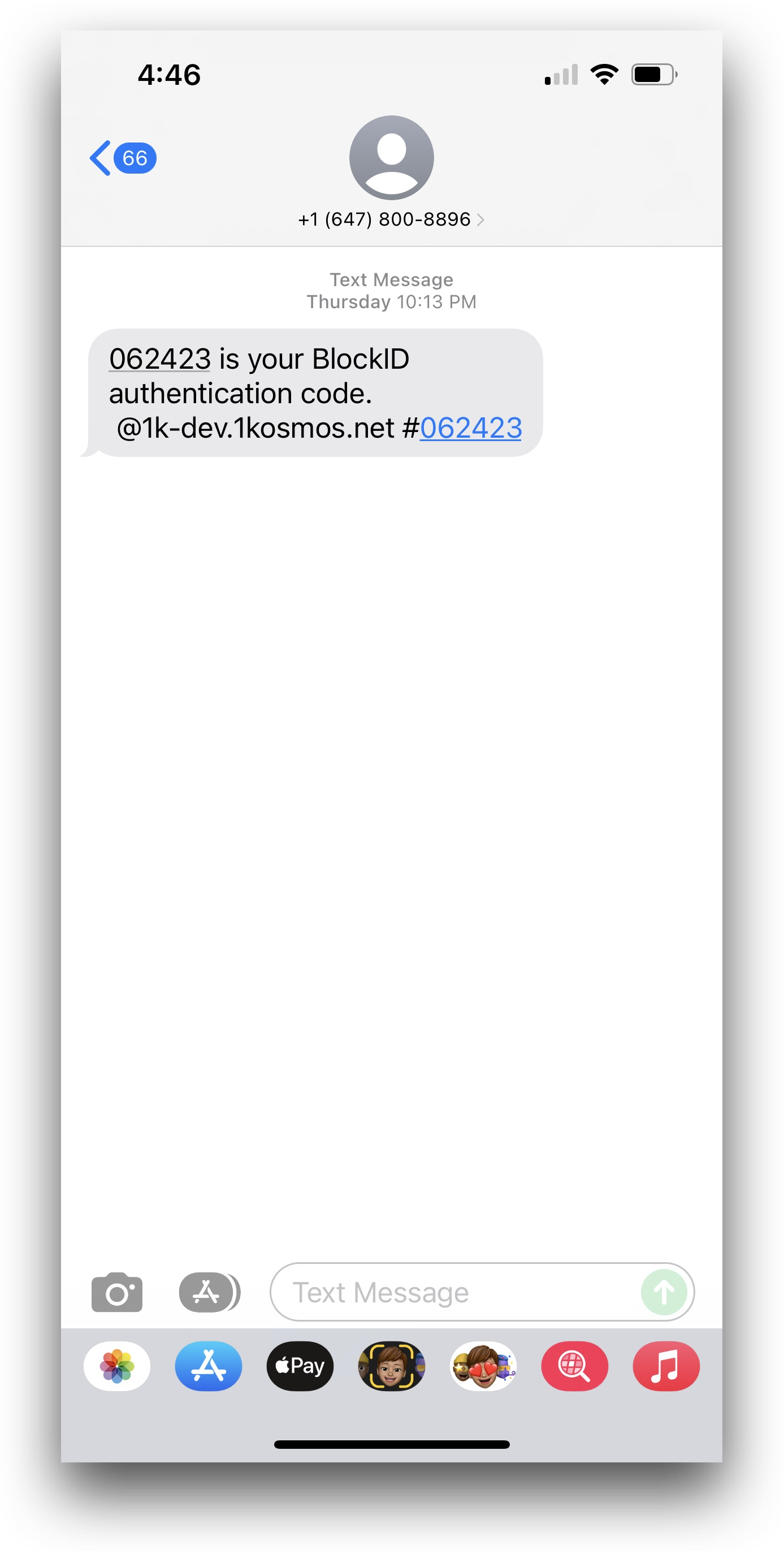
Example OTP Verifcation
BIDTenantInfo tenantInfo = new BIDTenantInfo("blockid-trial.1kosmos.net", "devx", "0005c9f8-1918-40be-aa00-e319043f7xxx");
BIDOtpVerifyResult result = BIDOTP.verifyOTP(tenantInfo, "john.smith@company.com", "062423");
Verify a one-time passcode request using the six digits you received via email or SMS.
Example OTP Request - SMS
Example SMS containing six-digit one-time passcode.
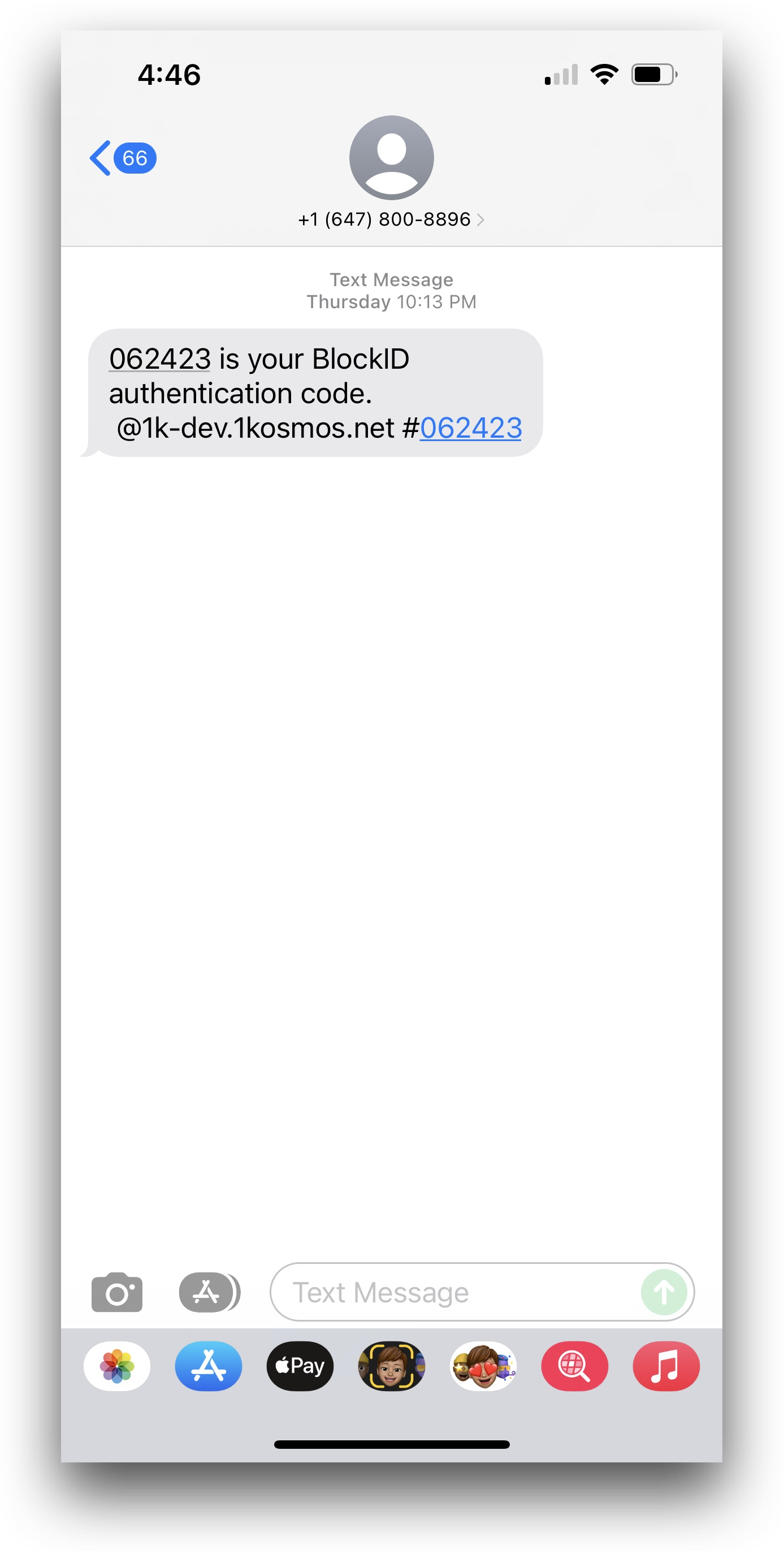
Example OTP Verifcation
using BIDHelpers.BIDOTP;
using BIDHelpers.BIDOTP.Model;
using BIDHelpers.BIDTenant.Model;
BIDTenantInfo bidTenantInfo = new BIDTenantInfo("blockid-trial.1kosmos.net", "devx", "0005c9f8-1918-40be-aa00-e319043f7xxx");
BIDOtpVerifyResult verifyOTP = BIDOTP.VerifyOTP(bidTenantInfo, "john.smith@company.com", "062423");
Verify a request with the six-digit one-time passcode you received via SMS or email.
Example Server Responses
The SDK will return an immediate response from our API. The responses are the same across each SDK and are JSON formatted. Possible response codes you might receive include:
Server Response
- 200
- 401
- 403
- 404
- 409
- 410
{
"messageId":"15f9dd86-9a8a-4c66-900a-f711f5acceb1",
"info":"OTP request verified"
}
The one-time passcode was verified and accepted.
{
"error_code": 401,
"message":"Unauthorized"
}
The request was not authorized.
{
"error_code": 403,
"message":"OTP locked for the user"
}
The incorrect one-time passcode was entered more than five times in 24 hours, resulting in an account lockout. User accounts will automatically unlock after 30 minutes.
{
"error_code": 404,
"message":"OTP is Invalid"
}
The API received an incorrect one-time passcode.
{
"error_code": 409,
"message":"OTP is already used"
}
The user already used the provided one-time passcode.
{
"error_code": 410,
"message":"OTP match found, but the validity time has expired"
}
The user entered a valid one-time passcode, but the validation time frame has expired.